OpenGUI is a high-Level C/C++ graphics & windowing library built upon a fast,
low-level x86 asm graphics kernel. OpenGUI provides 2D drawing primitives
and an event-driven windowing API for easy application development, and it supports the
BMP image file format. It's very powerful, but very easy to use. You can write apps in the
old Borland BGI style or in a windowed style like QT. OpenGUI supports
the keyboard and mouse as event sources, and the Linux framebuffer/svgalib/X11/DGA as
drawing backends. Mesa3D is also supported under Linux & Windows. On now 8, 15, 16 and
32-bit color modes are available.It is under LGPL license.
OpenGUI
is a very fast multi-platform 32-bit graphics library/GUI for MS-DOS (DPMI client/DJGPP),
QNX4 (Watcom or GCC), Windows and LINUX. It can be used to create graphic applications and
games for these Operating Systems. The library can be used with GNU C++ (from ver. 2.7.2)
or Watcom C++ (from 9.5) and NASM assembler (0.98). The library is very stable and is
ready for programs that require realtime drawing and Windowed GUI.
INHERITANCE DIAGRAM for DrawBuffer class
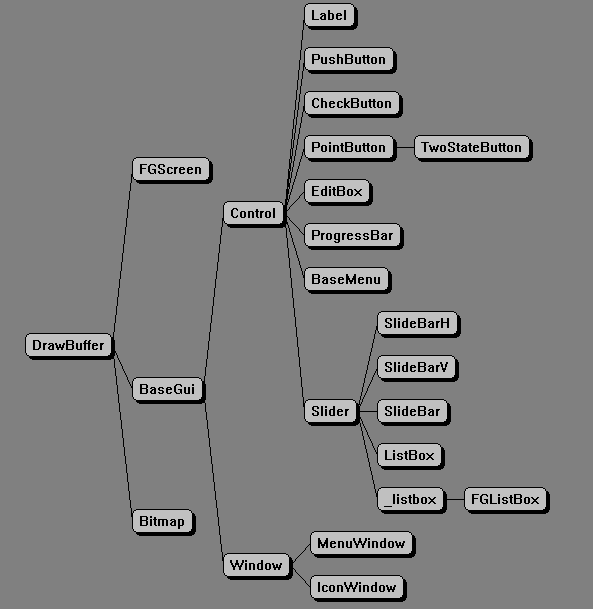
Controls tree
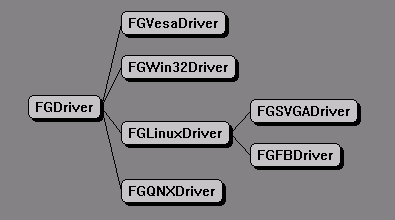
Basic driver/architecture configuration
(update: later was added Linux/X11 and SOLARIS 8 drivers)
Introduction - [slovak
version]
Features:
- LGPL
- ultra-fast (asm kernel & MMX support)
- the most robust library for the Linux FrameBuffer
- support for resolutions ranging from 320x200 to 1600x1200
- and 8, 15, 16 and 32 color depths
- windowing system
- object-oriented multi-platform API (DJGPP, WATCOM, GCC, LINUX, QNX,
BORLAND C++)
- full application development framework (configuration file, file
& color dialogs, etc.)
- professionally tested & actively used for some years (see
examples)
- a tool for interactive code generation (paint & run)
- and much more
The library consists of
three layers. The first layer is a hand-coded and fast assembler kernel. This layer does
the biggest piece of hard work. The second layer implements the API for drawing graphics
primitives like lines, rectangles, circles etc. This layer is comparable to Borland BGI
API. The third layer is written with C++ and offers a complete object set for the GUI
developer. The third layer implements objects like input windows, buttons, menus, bitmaps
etc, with addition of integrated mouse & keyboards support.
Links to similar project:
- Portable Threads - Pth
- QpThread library -
C++ advanced thread manipulating (Unix, Win32) (NEW)
- svgalib - linux console
graphics standard
- MiniGUI - pretty good windowing
in MS WIN32 style ...
- MicroWindows -
another good windowing lib
- skyvideo - linux,
win32 graphics library
- SDL - Simple DirectMedia Layer
- linux, BeOS, DirectX ... very COOL
- g2
- X11 and WIN32 graphics library
- uniforms - the
complete GUI for DJGPP (uses allegro & DLX system)
- GGI - general graphics
interface for linux
- xfb - linux
framebuffer accelerator
- official RHIDE homepage (NEW -
fixed)
- NASM - Netwide ASseMbler
|